Web chat
Streamline web chat with Slack and Thena web app
Overview
Thena's web chat seamlessly integrates with Slack and the Thena web app, effortlessly bridging the gap between the web chat and your Slack workspace. It automatically routes customer chats from your web dashboard to your pre-configured Slack channel, empowering your team to respond directly from Slack. With bi-directional sync, you can manage all conversations effortlessly across multiple platforms. You can also effectively reply to webchat conversations in real-time from the Thena web app, ensuring seamless communication between web chat, Slack, and Thena.
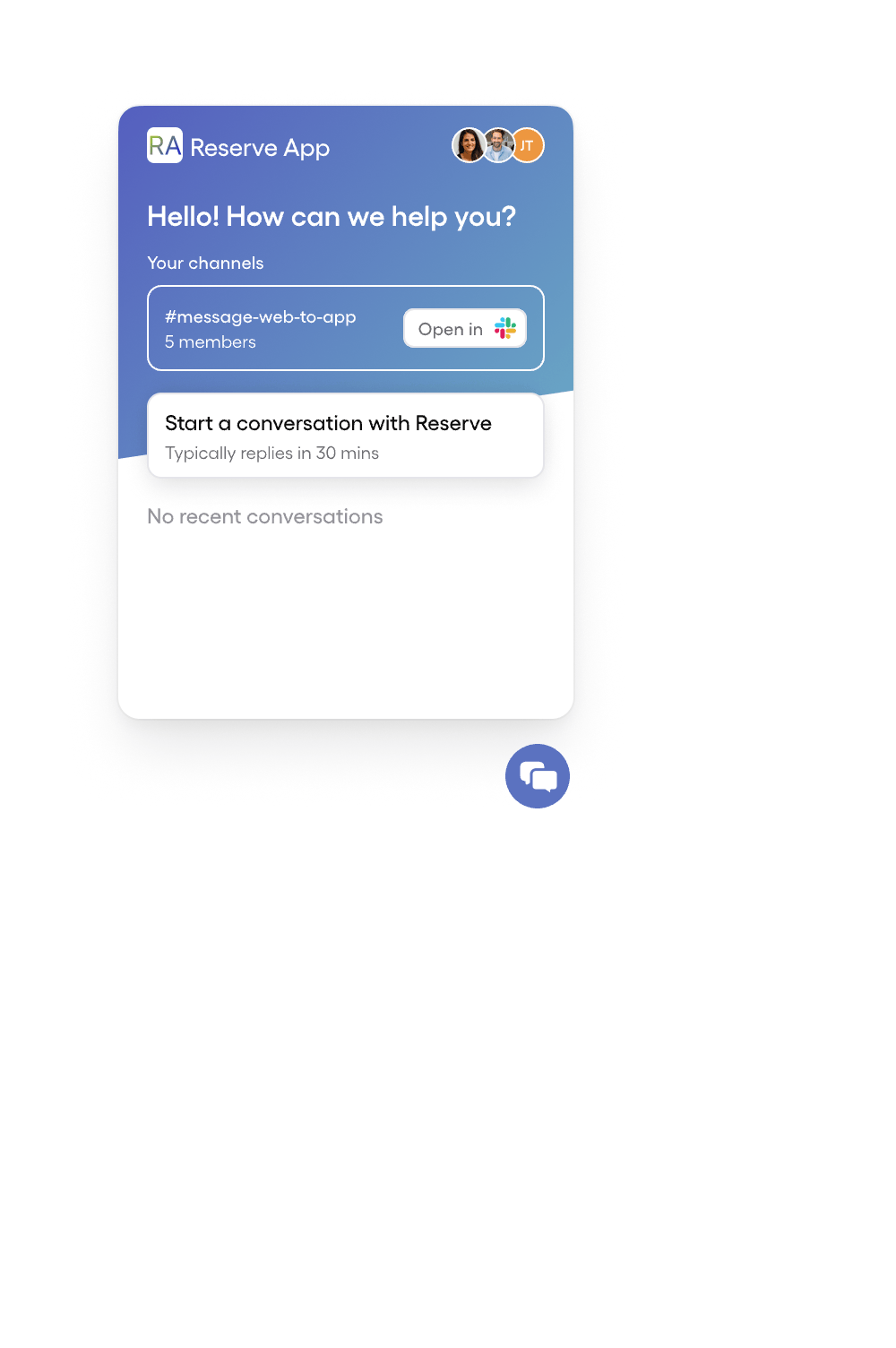
Features
Feature | Support |
---|---|
Route web chat messages to a Slack channel | ![]() |
Respond to web chat messages from Slack and Thena web app | ![]() |
Bi-directional sync of messages between Slack and web chat | ![]() |
See updated information in the web chat for messages that have been edited/deleted in Slack | ![]() |
Support for attachments and rich text | ![]() |
Manage web chat messages as Thena requests to assign, prioritize, and close | ![]() |
Edit & delete messages from web chat | Coming soon |
Embedded message reactions | Coming soon |
Pre-requisites
You will require the following:
- Designated Slack channel to receive and respond to messages from web chat
- The public domain where you want to implement web chat
- Access to your codebase for integrating the Thena web chat widget
- Parsed email of the user interacting with the web chat
We suggest setting up a new dedicated Slack channel for web chat integration
Configuration
Installing the web chat requires adding code to your frontend. You might need an engineer's help if you can't access the codebase.
Generate credentials
Navigate to Global Settings > Web Chat in the Thena web app
Setup domain whitelisting
Secure your services by only allowing trusted domains. By adding a domain to your whitelist, you permit interactions with your resources, guaranteeing that only approved domains can initiate requests to your platform. You have the option to include multiple domains, each added individually.
Enter only the domain names, excluding 'http://', 'https://', 'www' prefixes.
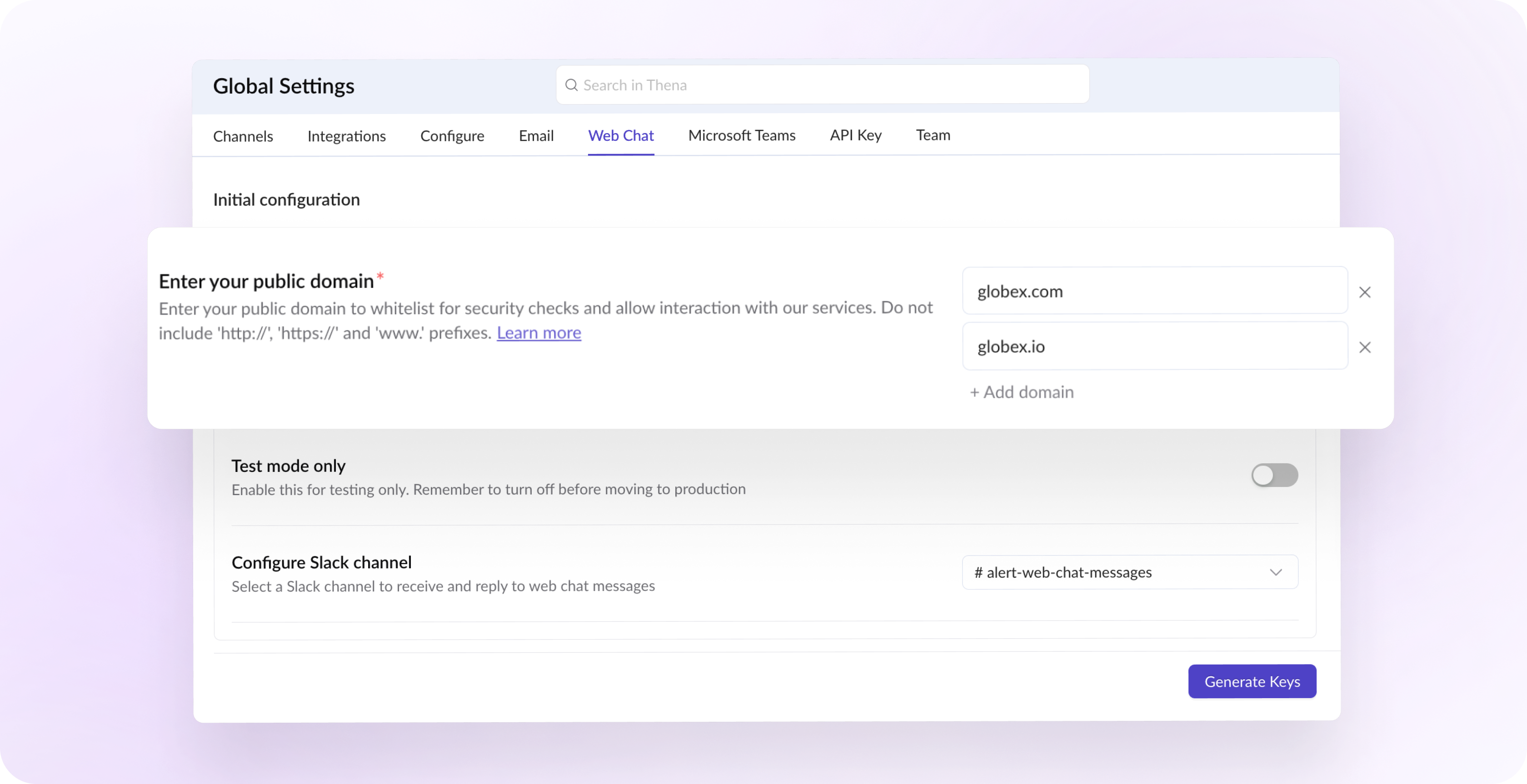
Enable Test mode
Enable the "Test mode only" toggle in order to test the web chat widget in your local servers.
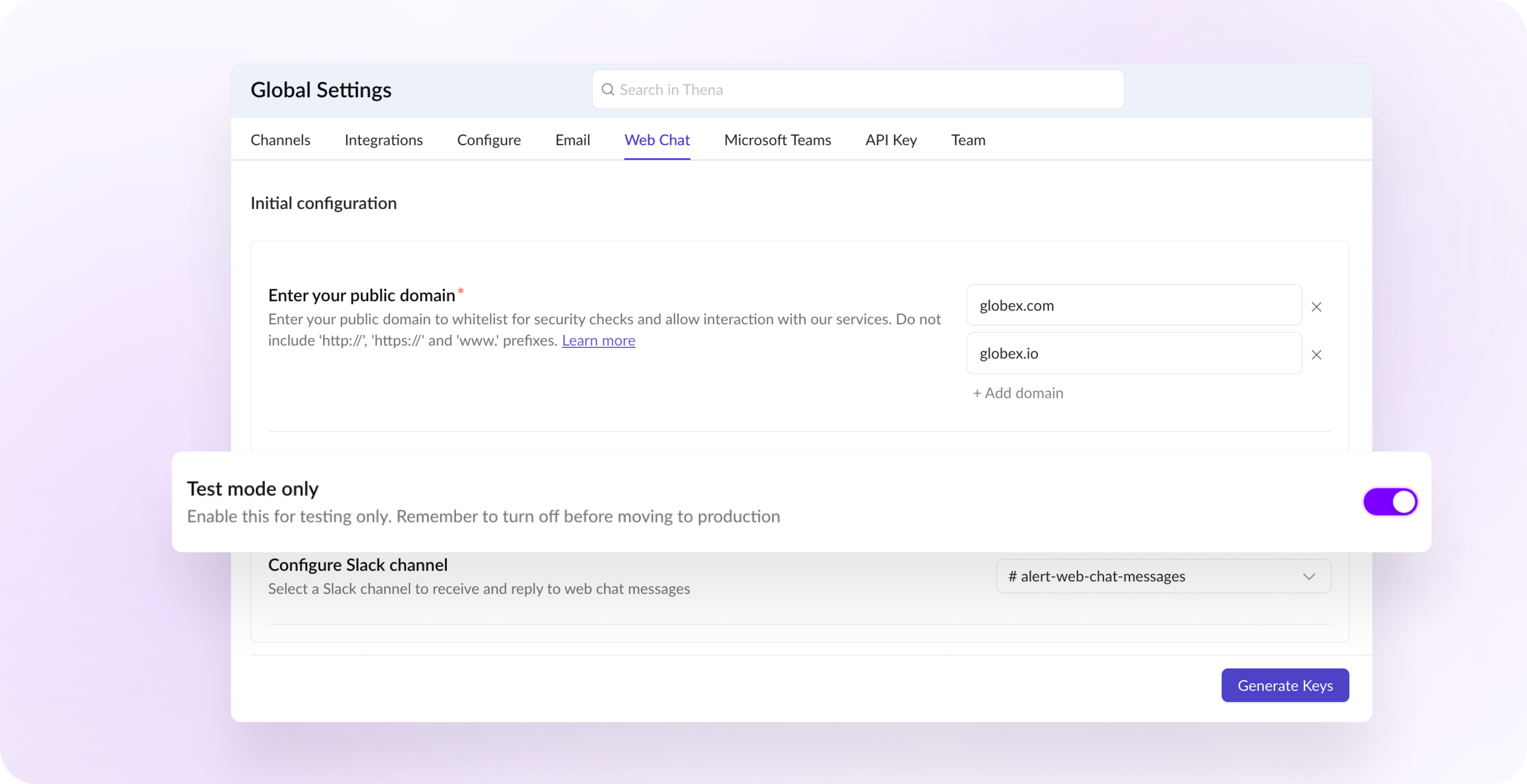
Note : "localhost" or "127.0.0.0" will be supported for testing only when the Test Mode toggle is enabled.
Setup Slack channel
Select the channel from the dropdown menu where you'd like to both receive and reply to web chat messages.
You can also switch the channel to a different one, at a later time.
Switching this channel will only mean updating the web chat communication channel. Your triage will continue to receive messages marked as Thena requests.
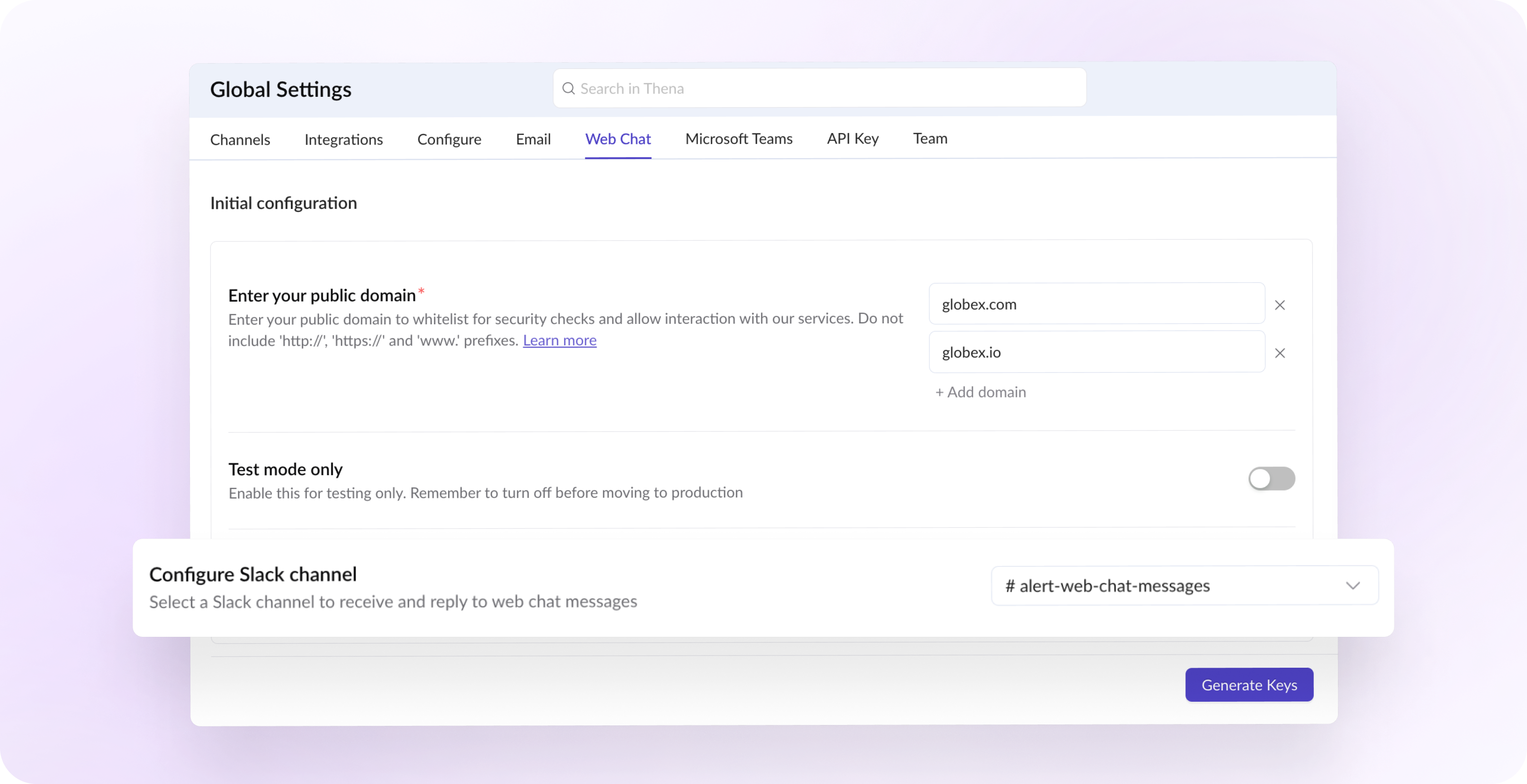
Switching your Slack channel will result in replies on the old channel not being sent on web chat. This happens because the web chat feature becomes associated with the newly configured channel.
Click on "Generate Keys", to receive the API Key
and Private Key
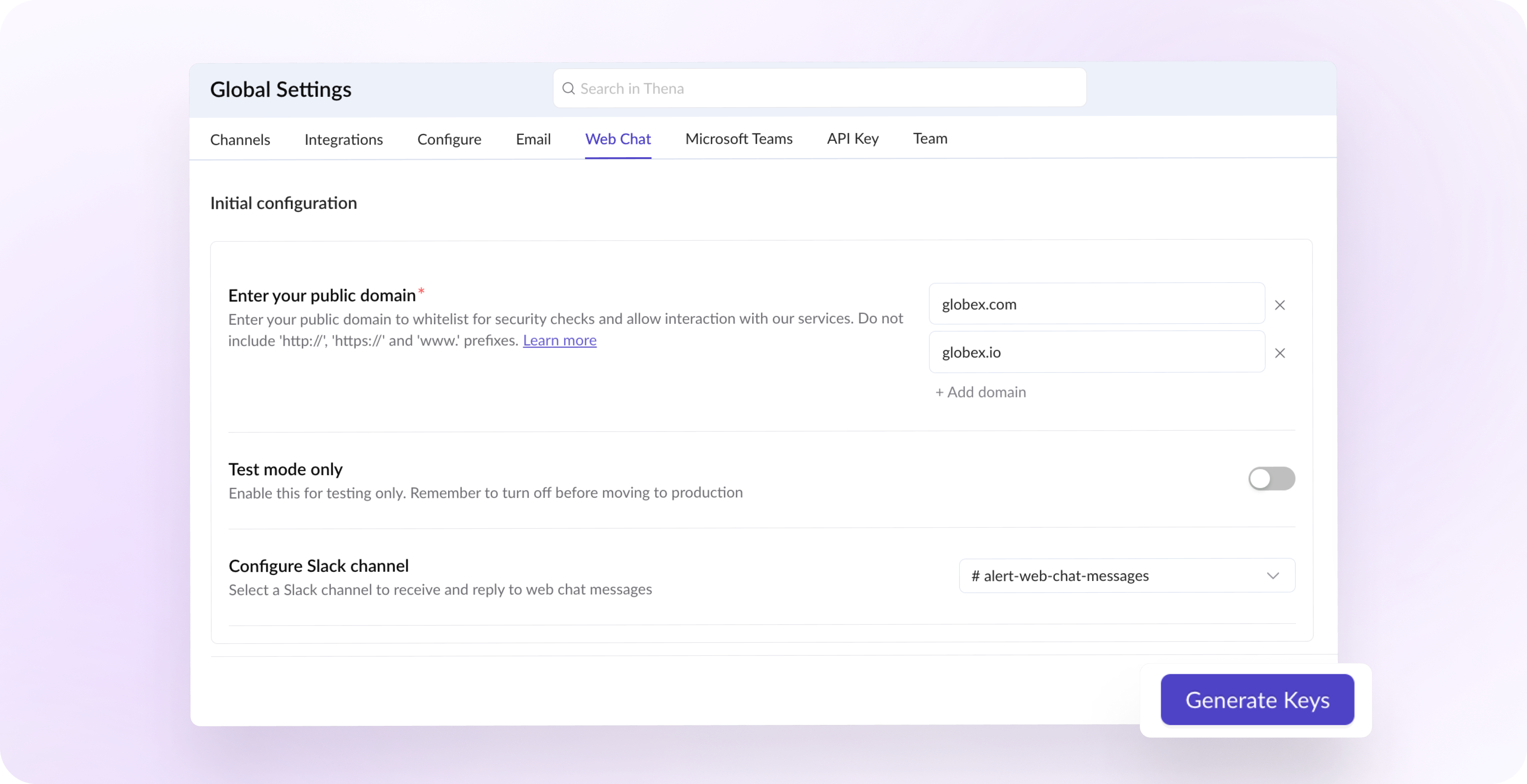
Once you have the generated API Key
and Private Key
, add these values in your frontend code as outlined below.
Add script to frontend code
Add the following code to your <HEAD>
tag in your index.html
file.
<script>
window.thenaWidget = {
thenaKey: 'API_KEY', // api key provided by Thena in the web-to-slack settings page
}
</script>
<script async>
;(function (window, document) {
;(window.thena = {}), (m = ['update', 'setEmail', 'logout'])
window.thena._c = []
m.forEach(
(me) =>
(window.thena[me] = function () {
window.thena._c.push([me, arguments])
})
)
var elt = document.createElement('script')
elt.type = 'text/javascript'
elt.async = true
elt.src = 'https://cdn.prd.cloud.thena.ai/shim.js'
elt.onload = function () {
window.thena.update(window.thenaWidget)
}
var before = document.getElementsByTagName('script')[0]
before.parentNode.insertBefore(elt, before)
})(window, document)
</script>
Enable the chat widget
You should add the below code wherever the user has already logged in and details like the userβs email become available.
useEffect(() => {
const init = async () => {
// call the hmac that you added in you backend
const hashedEmail = await yourFunctionToEncryptTheEmail(
'USER_EMAIL', // add user email here
'PRIVATE_KEY' // the private key provided by the thena in the web-chat setting page
)
// or just window.thena?.setEmail if not using TypeScript
(window as any).thena?.setEmail({
email: 'USER_EMAIL', // add user email here
hashedEmail: hashedEmail, // the hashed email returned by the hmac function
})
}
init()
}, [])
Thena mandatorily requires an email ID to authenticate the user on the web chat. It is recommended to hash the email id in order to ensure added security.
If the email is not parsed, the web chat widget will not be displayed
User identity verification
To ensure added security, implement identity verification for users sending messages via the chat widget. This prevents impersonation by prohibiting customers from manually altering their email addresses in the frontend.
In your backend, hash the userβs email address using HMAC-SHA256 with the Private_Key
generated in step 3.
It is mandatory to hash the email if you are not on test mode
const { createHmac } = require("crypto");
/**
* Generates an HMAC (Hash-based Message Authentication Code) using the provided email and private key.
* @param {Object} args - The args object.
* @param {string} args.email - The email to be used in the HMAC generation.
* @param {string} args.privateKey - The private key to be used in the HMAC generation.
* @returns {string} The generated HMAC.
*/
const generateHmac = ({ email, privateKey }) => {
return createHmac("sha256", privateKey).update(email).digest("hex");
};
const hashedEmail = generateHmac({
email: "USER_EMAIL",
privateKey: "PRIVATE_KEY",
});
console.log({ hashedEmail });
import hmac
import hashlib
def generate_hmac(email, private_key):
"""
Generate HMAC (Hash-based Message Authentication Code) for the given email and the private key.
Args:
email (str): The email address to generate HMAC for.
private_key (str): The private key used for HMAC generation.
Returns:
str: The HMAC generated for the email and private key.
"""
hmac_obj = hmac.new(private_key.encode(), email.encode(), hashlib.sha256)
hash_digest = hmac_obj.hexdigest()
return hash_digest
hashedEmail = generate_hmac(
email="USER_EMAIL",
private_key= "PRIVATE_KEY"
)
print('hashedEmail', hashedEmail)
The user is now verified when they open the chat
Appearance settings
Customize how the web chat looks to better align with your brand and user experience preferences.
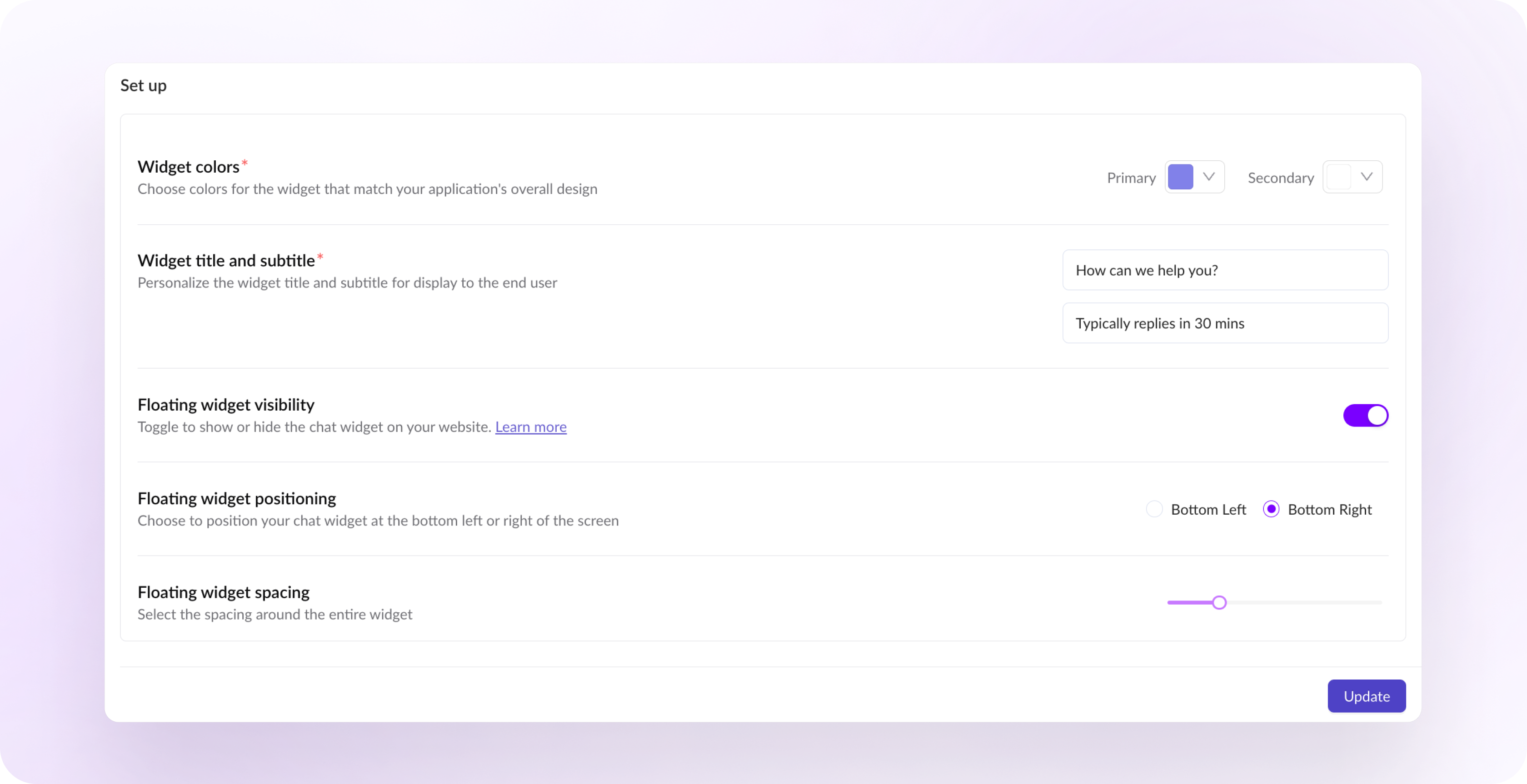
Widget customization | Comments |
---|---|
Colors | Select colors that enhance the appearance of your application |
Title/subtitle | Customize the messaging displayed to users in the widget's title and subtitle fields. |
Visibility | Choose to hide the widget on the application. |
Positioning | Customize the widget's location on the screen. Available options - bottom left/bottom right |
Spacing | Fine-tune the padding around the widget to better fit your screen layout |
Toggle widget
The widget is activated when the floating action button is clicked. However, you have the flexibility to programmatically open or close the web chat widget based on specific requirements, such as a button click event. This can be achieved using the following code snippet, allowing for customized interaction within your application.
function toggleThenaWidget() {
const thenaIframeEle = window.document.getElementById(
'thena-iframe-element'
)
thenaIframeEle?.contentDocument?.dispatchEvent(
new CustomEvent('TOGGLE_THENA_WIDGET')
)
}
// call this function from your UI to toggle the widget
Use cases
Web chat <> Slack - Bidirectional conversation flow
Once the setup is configured, web chats will be forwarded to the configured Slack channel. Your support agents can reply to the web chat from Slack, and your customer will receive the response on web widget where they originally reached out.
Formats | Web chat to Slack | Slack to web chat | Thena Web app to web chat |
---|---|---|---|
Plain text | ![]() | ![]() | ![]() |
Italic text | Coming soon | Coming soon | Coming soon |
Bold text | Coming soon | Coming soon | Coming soon |
Strikethrough | Coming soon | ![]() | Coming soon |
Embedded hyperlinks | ![]() | ![]() | ![]() |
Embedded code | Coming soon | ![]() | Coming soon |
Attachments | ![]() | ![]() | ![]() |
Bullets, Numbering | Coming soon | ![]() | Coming soon |
Emoticons | ![]() | ![]() | ![]() |
Web chats as Thena Requests
Thena routes the web chat messages to your configured Slack channel identifying them as requests and send internal notifications on the configured triage channel.
After receiving the request, you have the ability to perform standard actions such as adjusting its priority, responding, assigning it, and more.
FAQs
Can any of my customers use Thena web chat on my dashboard?
Any customer or user who has their email parsed on your website or dashboard can begin using the Thena web chat.
Can I choose where to send the customer messages?
You can send customer messages to the Slack channel configured while setting up web chat. This can also be updated later. However, replies on all existing messages on the previously configured Slack channel cannot be received by the web chat users.
Can I reply directly to the Slack thread to start chatting with the customer?
Yes, once the message comes from the web chat, you can simply reply on that message thread to continue to communication. Any further messages from the same user will be part of the same Slack thread.
Can we restrict web chat to only show for certain customers?
No, Thena will not be able to restrict who sees the plugin as of today. To limit web chat from showing for certain customers, you can block the web SDK from loading for the customers that you want to restrict.
Are CSATs currently supported in the Web Chat product?
No, CSATs are currently not supported in the Web Chat product
What is the approximate size of the web chat SDK?
The web chat SDK is 324KB
Updated 3 days ago